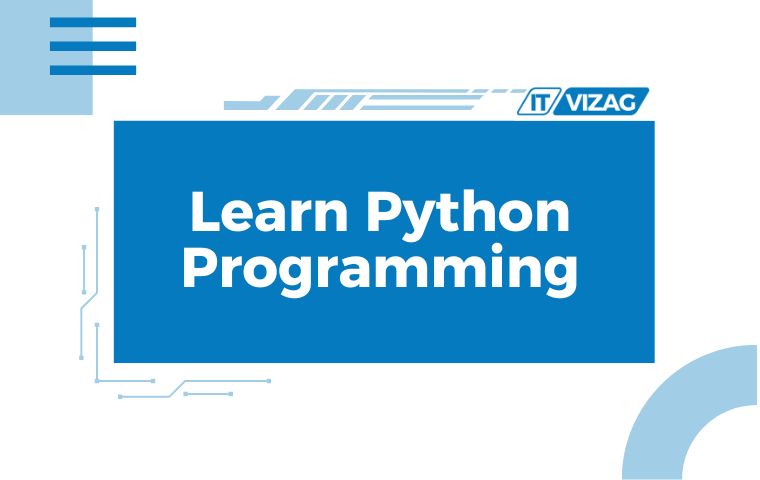
About Course
This course introduces students to the world of programming using Python, a versatile and beginner-friendly language. By the end of the course, students will understand the basic concepts of Python and be able to write simple programs. This course is designed for absolute beginners, making it ideal for students new to coding.
Course Objectives
By completing this course, students will:
- Understand the basics of Python programming.
- Learn how to use variables, data types, and operators.
- Create programs using conditional statements and loops.
- Explore functions and their importance in programming.
- Gain confidence to pursue further learning in programming.
Course Content
Module 1: Introduction to Python
- What is Python, and Why Learn It?
- Installing Python and Setting Up the Environment
- Writing and Running Your First Python Program
- Congratulations
Module 2: Variables and Data Types
- Understanding Variables
- Common Data Types in Python
- Performing Basic Operations with Data Types
- String Operations
- Boolean Operations
- Hands-On Practice
Module 3: Conditional Statements
Introduction to Conditional Statements
- Introduction to Conditional Statements
- Basic If-Else Statement
- Using Elif for Multiple Conditions
- Comparison Operators
- Logical Operators
- Nested If Statements
- Practical Examples
- Hands-On Practice
Module 4: Loops in Python
- Introduction to Loops
- For Loops
- While Loops
- Combining Loops with Conditional Statements
- Fun Exercise: Loops with Emojis
- Hands-On Practice
Module 5: Functions in Python
- Introduction to Functions
- Defining and Calling Functions
- Function Parameters and Arguments
- Return Statement
- Fun Example: Making a Calculator
- Default Parameters
- Fun Example: Emoji Decorator Function
- Lambda Functions
- Fun Example: Random Joke Generator
- Hands-On Practice
Module 6: Lists and Tuples in Python
- Introduction to Lists and Tuples
- Understanding Lists
- Fun Example: Random Team Generator
- Understanding Tuples
- Fun Example: Emoji Coordinates
- Hands-On Practice
Module 7: Dictionaries and Sets in Python
- Introduction to Dictionaries and Sets
- Understanding Dictionaries
- Fun Example: Word Counter
- Understanding Sets
- Fun Example: Unique Letters Finder
- Hands-On Practice
Module 8: File Handling in Python
- Introduction to File Handling
- Opening and Closing Files
- Writing to a File
- Reading from a File
- Appending to a File
- Using the with Statement
- Handling File Errors
- Fun Example: Diary App
- Hands-On Practice
Module 9: Error and Exception Handling in Python
- Introduction to Error Handling
- Errors vs. Exceptions
- Handling Exceptions with try and except
- The finally Block
- Raising Exceptions
- Common Python Exceptions
- Fun Example: Simple Calculator with Error Handling
- Hands-On Practice
Module 10: Advanced Python Concepts
- Introduction to Advanced Python
- Generators and Iterators
- Decorators
- Context Managers
- Regular Expressions
- Hands-On Practice
Module 11: Automation with Python
- Introduction to Automation
- Automating Files and Folders
- Web Scraping
- Sending Emails with Python
- Automating Excel Tasks
- Hands-On Practice
Module 12: Data Visualization with Python
- Introduction to Data Visualization
- Getting Started with Matplotlib
- Advanced Visualizations with Seaborn
- Interactive Visualizations with Plotly
- Combining Visualizations
- Hands-On Practice
Module 13: Advanced Topics in Python
- Exploring Advanced Python Features
- Decorators
- Generators
- Context Managers
- Multi-threading and Multi-processing
- Creating and Using Python Packages
- Hands-On Practice